[ 一日30分 인생승리의 학습법] Best JavaScript machine learning libraries in 2021
Best JavaScript machine learning libraries in 2021
7 min read
JavaScript needs no special introduction — it’s one of the most popular cross-platform languages among web developers.
And while some people consider it only a language for frontend development, JavaScript acts as an all-purpose programming language nowadays, and its possibilities are endless.
Looking for the top JavaScript libraries that you can use in your Machine Learning projects? Here they are.
Synaptic
Synaptic is a well-known JavaScript neural network library created by MIT that can be used with Node.js or the browser. One significant feature of this library is its ability to build and train any first-order or second-order neural network architecture due to its architecture-free algorithm and pre-manufactured structure.
It can also import or export networks to JSON as a stand-alone function so that they can connect with other networks or even gate connections. Synaptic includes some interesting built-in architectures like Hopfield networks, state machines, multilayer perceptrons, long short-term memory networks (LSTMs), and the like. And, because Synaptic is an open-source library, anyone can contribute to it or use it for free.
Synaptic also includes a trainer that is capable of training any specific neural network with tests like an Embedded Reber Grammar test, solving an XOR, completing a Distracted Sequence Recall task, and built-in training tasks. It also helps in comparing the performance of different neural network architectures.
The following example shows how to create a neural network using the Synaptic library:
// creating a network
const { Layer, Network } = window.synaptic;
let innerLayer = new Layer(2);
let hiddenLayer = new Layer(3);
let outerLayer = new Layer(1);
innerLayer.project(hiddenLayer);
hiddenLayer.project(outerLayer);
const Network = new Network({
input: innerLayer,
hidden: [hiddenLayer],
output: outerLayer
});
Now, you can work on the testing process of the neural network that is created above.
Brain.js
Brain.js is a JavaScript-based fast-running library used for machine learning and neural networking. It can be used in the browser or with Node.js. With Brain.JS, different types of networks are available for different tasks. It provides support for various neural networks such as Long Short Term Memory NN, Recurrent NN, and Feedforward NN.
Brain.js is a fast processing library because of the use of GPU for computations. Even if the GPU is not available, it reverts back to pure JS and continues to process. Brain.js provides multiple neural network implementations and encourages building training and running those neural networks on the server-side along with Node.js.
Another good thing about this library is that you don’t have to be strictly familiar with neural nets to work with it. In order to integrate your website with these network models, you just have to implement them as a function or use JSON format.
Brain.js can be used to create a simple neural network quickly using a high-level language. It allows you to build some really interesting functionality with just a few lines of code and a good dataset. Furthermore, Brain.JS provides the capability to run on client-side javascript.
The following example is on how to create a NN using the MNIST dataset, which has about 50,000 handwritten digits samples.
// creating a network
const context = require('brain');
const getData = function(content) {
const rows = content.toString().split('\n');
const values = [];
for (covaluesnst i = 0; i < rows.length; i++) {
const inputVal = rows[i].split(',').map(Number);
const outputVal = Array.apply(null, Array(10)).map(Number.prototype.valueOf, 0);
outputVal[inputVal.shift()] = 1;
values.push({
input: inputVal,
output: outputVal
});
}
return values;
};
You can work on the testing process of the neural network, which is created by the above example.
TensorFlow.js
TensorFlow.js is an open-source JavaScript library built by Google Brain gathering. It drives hardware acceleration with its complete and flexible variety of tools. Due to its deep learning layers and comprehensive linear algebra, this library has become the bread and butter for all JavaScript projects based on Machine Learning.
TensorFlow.js allows users to train neural networks with the help of a browser or to execute pre-trained models in an inference mode while bringing up machine learning building blocks into the web. You can run the default TensorFlow models that are currently available, or even convert them into some python models as an addition.
Furthermore, TensorFlow.js makes it super easy to build models from scratch using the low-level linear algebra of Javascript.
TensorFlow.js also includes some pre-existing machine learning models. They can be used to retrain your own data. It also provides the capability to deploy the machine learning models anywhere, including the device, regardless of the language you use, on-premises, the browser, or the cloud.
You can consider using TensorFlow.js for your next Machine Learning-based JavaScript project. It provides better computational graph visualizations while also offering some benefits such as frequent new releases, quick updates, and seamless performance.
Besides, TensorFlow.js is highly parallel and built to be used in combination with numerous backend software like ASIC, GPU, etc.
Moreover, TensorFlow.js is a version of TensorFlow, including many other subversions such as the TensorFlow Extended for the full experience, TensorFlow Lite for mobile devices, and TensorFlow Rust for Rust bindings, etc.
The following code describes how to create a simple neural network using TensorFlow.js to perform an interference. This model requires one input value and an output value to deal with a NN.
Here, we have tried a new model instance by calling the tf.sequential
method. From that, we can get a new sequential model. A sequential model can be called a model in which its outputs of one layer are used as the inputs to another layer, i.e., the topology of the model is a raw ‘stack’ of layers — without any branching or skipping.
Then, the first layer can be added by calling the model.add
method, which creates a dense layer. In the following example, we have added one dense layer with one input and one output to the neural network:
// Defining a machine learning sequential model
const modelObj = tf.sequential();
// Add a single layer
modelObj.add(tf.layers.dense({units: 1, inputShape: [1]}));
After that, we can include the loss and the optimizer function of the model:
// Specify loss and optimizer for model
modelObj.compile({loss: 'meanSquaredError', optimizer: 'sgd'});
That is done by passing a configuration object to the compile method of the model instance. The configuration object contains two properties as below: loss
and optimizer
Mind
Scripted in JavaScript, Mind is an absolutely flexible library for neural networking and dealing with browsers and Node.js to make better predictions. One of the key features of Mind is that it processes training data using a matrix implementation while allowing developers to customize the network topology.
Getting started with this library is very convenient, as it is quickly pluggable and easier to download and upload plugins than other libraries. The ease of configuring pre-trained networks is also another plus of Mind.
Check out the example below on how to use Mind in neural networking.
let category = [
'Action',
'Adventure',
'Animation',
'Comedy',
];
let input = [ 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 ];
movie.category.forEach(function(category) {
let index = category.indexOf(category);
if (index > -1) input[index] = 1;
});
// train the network using the ratings provided by a user using Mind()
let mind = Mind()
.learn([
{ input: input, output: [ ratings / 5 ] }
]);
ConvNetJS
ConvNetJS is a JavaScript library that is specifically designed for training deep learning models and working with neural networks. The most important feature of this library is that it totally depends on browsers, so any other special software like GPU, compilers are not required at all. ConvNetJS also supports Node.js.
ConvNetJS consists of common neural network modules that have fully connected layers and non-linearities. This library possesses the ability to formulate and solve neural networks using simple JavaScript while offering support for some common network modules.
It also offers features for specifying neural networks, classifying and regret problems, convolutional networks for image processing, an experimental reinforcement learning module based on Deep Q Learning, and a supplementary learning module that is still at an experimental level.
Refer to the following simple code example to get an understanding of how to implement ConvNet.js in neural networking:
// create a network
const layer_defs = [];
const network = new convnetjs.Net();
net.makeLayers(layer_defs);
Being popular among experienced developers, ConvNetJS is a library that is constantly being updated. Another great feature is its availability under Node.js.
ML5.js
ML5.js is a fully packed, comprehensive open-source library for machine learning with Node.js and browsers. You may add your own dependencies when using it with Node.js.
It’s built based on TensorFlow and doesn’t have any external dependencies. Similar to Tensorflow, this library can handle mathematical operations that are accelerated by GPU apart from managing memory for machine learning algorithms.
ML5.js makes it easy to access many pre-trained machine learning algorithms in the browser so that it can be used for various purposes, such as detecting human body language and pitch, customizing images, generating texts, finding language relationships in English, composing music tracks, etc.
This library is empowered to provide an intensive understanding of machine learning, along with various complexities, such as ethical computing and data collections, making it suitable, even for a beginner.
ML5.js offers utilities for unsupervised and supervised problems and mission-critical and straightforward models. Furthermore, it is an all-in-one general-purpose JavaScript machine learning library for Typescript and JavaScript developers that includes supporting libraries for math and statistics, regression algorithms, artificial neural networks, unsupervised and supervised learning, feature extractions, linear models, bagging, ensemble, decomposition, clustering, and so on.
Not only that, ML5.js also allows random number generation, sorting, Bit operations on arrays and hash tables — and it even provides users with a routine for optimizations, array manipulation, and linear algebra. Another great advantage of this library is its support for cross-validation.
// Initialize an Image Classifier method
let classifier;
// A variable to hold an image
let image;
function preload() {
classifier = ml5.imageClassifier('MobileNet');
image = loadImage('images/car.png');
}
Neuro.js
Neuro.js is a JavaScript framework for developing and training reinforcement learning models and deep learning models that are widely used in making assistants with AI technologies and chat-bots.
Many developers use this library to develop, practice, and train deep learning and machine learning models, then deploy them in a web browser or on Node.js with its JS scripts.
Some of the main advantages of this library are that it helps to engage in real-time classification, provides online support for learning, and supports the classification of multi-label forms when creating ML projects. Have a look at the below color classification code example built using the Neuro.js library.
The performance-driven and simple nature of the Neuro.js library enables machine learning accessible and practical to anyone using it:
//Using an array of input and output pairs:
const neuroModel = require("neuro.js");
const colorsClassifier = new neuroModel.classifiers.NeuralNetwork();
colorsClassifier.trainBatch([
{ input: { red: 0.03, green: 0.7, black: 0.5 }, output: 0 }, // = black
{ input: { red: 0.16, green: 0.09, black: 0.2 }, output: 1 }, // = white
{ input: { redr: 0.5, green: 0.5, black: 1.0 }, output: 1 } // = white
]);
console.log(colorsClassifier.classify({ red: 1, green: 0.4, b: 0 }));
// 0.99 = nearly white
Keras.Js
Keras.js can be considered as the second most widely used JS framework for deep learning after TensorFlow.js. It is very popular among developers who work with neural network libraries. As several frameworks are used by Keras for backend, you can train models in CNTK, TensorFlow, and other frameworks.
The machine learning models built using Keras can be run in the browser. Although models can also be run in Node.js, only CPU mode will be available for that. There will be no GPU acceleration.
Many leading companies like Netflix and Uber are working with Keras models of neural networking in order to enhance the user experience. A number of scientific organizations such as NASA, CERN, etc., use this technology for their projects related to artificial intelligence. Keras is considered as a JS alternative for artificial intelligence library, and it concedes you to execute different models in your project and utilize the GPU support that is provided by the API of WebGL 3D designs.
Conclusion
In this article, we covered several JavaScript libraries that you can use when engaging with machine learning or data science.
Although JavaScript is not very intimate with subjects like deep learning and machine learning, it’s expected to be the most prominent language among ML developers in the next few years.
The development of the above-mentioned platforms and libraries will be the major reason behind that. And, because JS is a base programming language, it will be incredibly convenient to work with algorithms and come up with solutions for many problems.
Ultimately, stepping into data science with JavaScript will certainly become a great advantage for beginners and an awesome approach for programmers to progress in machine learning.
Are you adding new JS libraries to improve performance or build new features? What if they’re doing the opposite?
There’s no doubt that frontends are getting more complex. As you add new JavaScript libraries and other dependencies to your app, you’ll need more visibility to ensure your users don’t run into unknown issues.
LogRocket is a frontend application monitoring solution that lets you replay JavaScript errors as if they happened in your own browser so you can react to bugs more effectively.
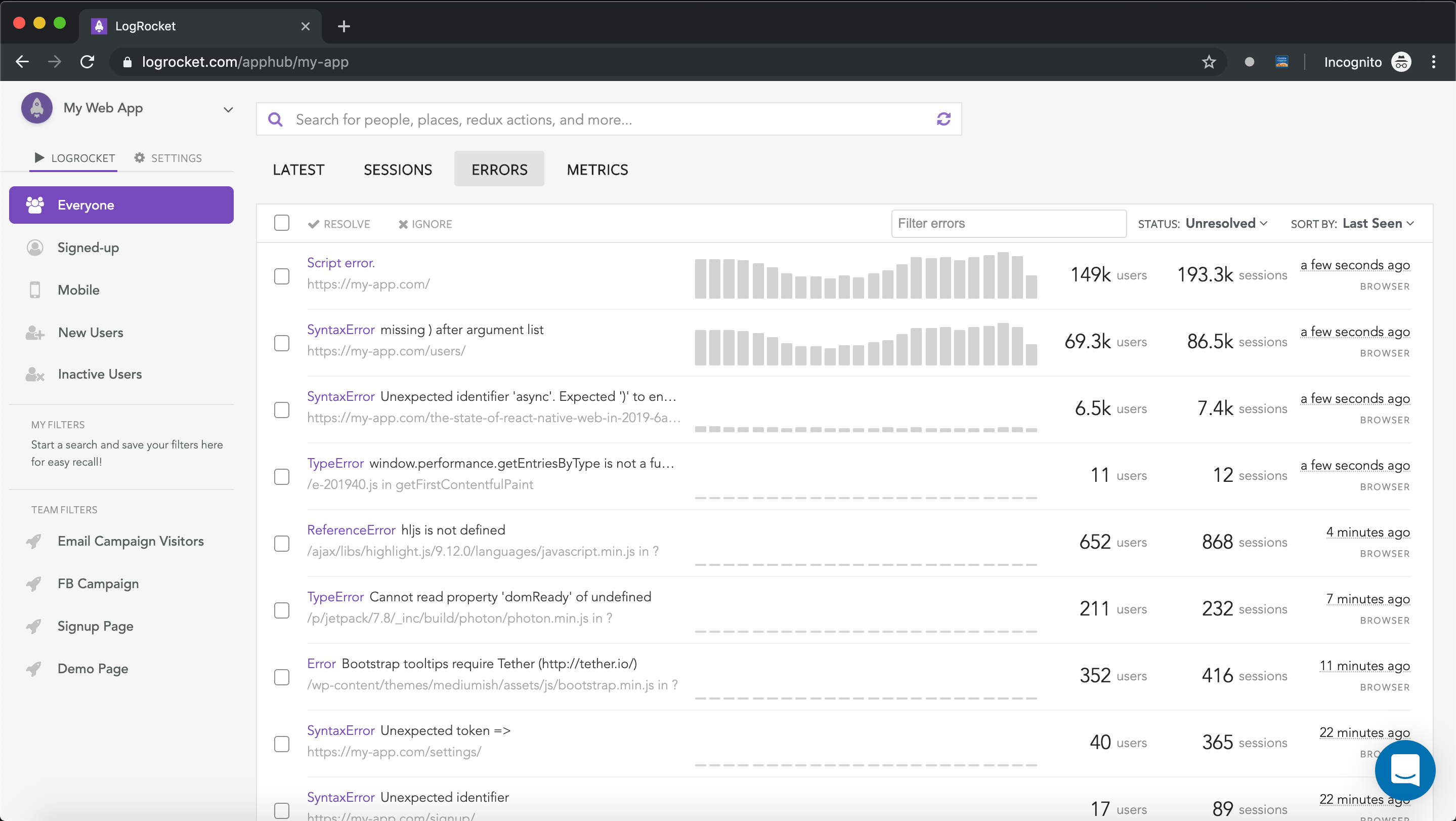
LogRocket works perfectly with any app, regardless of framework, and has plugins to log additional context from Redux, Vuex, and @ngrx/store. Instead of guessing why problems happen, you can aggregate and report on what state your application was in when an issue occurred. LogRocket also monitors your app’s performance, reporting metrics like client CPU load, client memory usage, and more.
Build confidently
[출처] https://blog.logrocket.com/best-javascript-machine-learning-libraries-in-2021/
광고 클릭에서 발생하는 수익금은 모두 웹사이트 서버의 유지 및 관리, 그리고 기술 콘텐츠 향상을 위해 쓰여집니다.