- 전체
- Native Apps
- WinJS App
- C# Apps
- XAML
- VB.net
- VisualC.net
- C++
- MFC
- visual studio mobile app dev
- Azure ms cloud service
- Asp.net
- 인공지능 (AI)
- wpf
- UWP
- MAUI
- asp.net
C# Apps [C# app] Pythonnet – .NET Core와 Python의 간단한 결합 : Pythonnet – A Simple Union of .NET Core and Python You’ll Love
2023.03.11 21:33
[C# app] Pythonnet – .NET Core와 Python의 간단한 결합 :
Pythonnet – A Simple Union of .NET Core and Python You’ll Love
Pythonnet – .NET Core와 Python의 간단한 결합
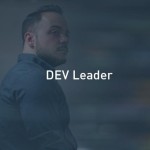
Python은 점점 인기를 얻고 있는 강력하고 다재다능한 프로그래밍 언어입니다. 많은 사람들이 시작할 때 선택하는 첫 번째 프로그래밍 언어 중 하나입니다. 몇 년이 지난 후 내 블로그에서 가장 트래픽이 많은 게시물 중 일부는 C#과 Python을 함께 사용하는 방법을 살펴봅니다. 오늘, 우리는 C# .NET Core 애플리케이션 내에서 내 원래 기사 보다 훨씬 더 현대적인 접근 방식으로 Python을 사용하는 방법을 탐색할 것입니다 . 파이썬넷에 들어가세요!
Pythonnet 패키지 및 시작하기
우리는 이 목표를 달성하기 위해 Python for .NET을 살펴볼 것입니다 . 이 라이브러리를 사용하면 .NET Core 애플리케이션 내에서 실행 중인 컴퓨터에 설치된 Python을 활용할 수 있습니다. 사용하려는 해당 Python DLL을 가리키도록 구성해야 하며 몇 줄의 초기화 후에 경주를 시작합니다!
예제 1 – Pythonnet을 사용한 Hello World
시작하려면 NuGet 에서 pythonnet 패키지를 설치해야 합니다 . 완료하면 다음 코드를 사용하여 C# 코드에서 Python 스크립트를 실행할 수 있습니다.
using Python.Runtime; internal sealed class Program { private static void Main(string[] args) { // NOTE: set this based on your python install. this will resolve from // your PATH environment variable as well. Runtime.PythonDLL = "python310.dll"; PythonEngine.Initialize(); using (Py.GIL()) { using var scope = Py.CreateScope(); scope.Exec("print('Hello World from Python!')"); } } }
이 코드는 런타임에서 Python DLL 경로를 설정하며 이는 필요한 단계입니다. 이것을 하는 것을 잊지 마세요 ! PythonEngine.Initialize()
그런 다음 and 를 호출해야 합니다 Py.GIL()
. 나중에 처리하고 싶으므로 명령문 을 고려하십시오 using
. static
Py
사용할 범위를 생성하도록 클래스에 요청한 다음 일부 Python 코드를 실행하기 위해 Exec 메서드를 활용할 수 있습니다 . 이 예제에서는 Exec 메서드를 호출하여 Hello World from Python!
콘솔에 " "를 인쇄하는 간단한 Python 스크립트를 실행합니다 .
예제 2 – Pythonnet 계산기!
Python C API를 사용하여 C# 코드에서 직접 Python 함수를 호출할 수도 있습니다. 이렇게 하려면 호출하려는 Python 함수에 대한 C# 래퍼를 만들어야 합니다. 다음은 두 개의 정수를 인수로 사용하고 그 합계를 반환하는 Python 함수에 대한 래퍼를 만드는 방법의 예입니다.
using System; using Python.Runtime; internal sealed class Program { private static void Main(string[] args) { // NOTE: set this based on your python install. this will resolve from // your PATH environment variable as well. Runtime.PythonDLL = "python310.dll"; PythonEngine.Initialize(); using (Py.GIL()) { // NOTE: this doesn't validate input Console.WriteLine("Enter first integer:"); var firstInt = int.Parse(Console.ReadLine()); Console.WriteLine("Enter second integer:"); var secondInt = int.Parse(Console.ReadLine()); using dynamic scope = Py.CreateScope(); scope.Exec("def add(a, b): return a + b"); var sum = scope.add(firstInt, secondInt); Console.WriteLine($"Sum: {sum}"); } } }
이 예에서는 Exec 메서드를 사용하여 add
두 개의 정수를 인수로 사용하고 해당 sum
. C#의 dynamic 키워드 덕분에 개체 에 직접 scope
호출되는 메서드가 있다고 가정할 수 있습니다 . 마지막으로 C# 내부에 있는 것처럼 add
C# 코드를 직접 사용하여 메서드를 호출합니다 . add
C#에서 변수 에 할당된 반환 유형 sum
도 동적이지만 이 변수를 정수로 선언할 수 있으며 이 유형으로도 제대로 컴파일됩니다.
예제 3 – 개체 상호 운용성
Python 개체를 C# 함수에 전달할 수도 있고 그 반대의 경우도 가능합니다. 다음은 Python 목록을 C# 함수로 다시 수신하고 dynamic 키워드를 사용하지 않고 해당 요소를 반복하는 방법에 대한 예입니다.
using Python.Runtime; internal sealed class Program { private static void Main(string[] args) { // NOTE: Set this based on your Python install. // This will resolve from your PATH environment variable as well. Runtime.PythonDLL = "python310.dll"; PythonEngine.Initialize(); using (Py.GIL()) { using var scope = Py.CreateScope(); scope.Exec("number_list = [1, 2, 3, 4, 5]"); var pythonListObj = scope.Eval("number_list"); var csharpListObj = pythonListObj.As<int[]>(); Console.WriteLine("The numbers from python are:"); foreach (var value in csharpListObj) { Console.WriteLine(value); } Console.WriteLine("Press enter to exit."); Console.ReadLine(); } } }
이 예제에서는 Exec 메서드를 사용하여 Python 목록을 만들고 이라는 변수에 할당합니다 number_list
. 그런 다음 Eval 메서드를 사용하여 개체 에 대한 참조를 가져온 list
다음 As<T>
정수 배열( 로 표시됨 int[]
)을 호출하여 결과를 변환할 수 있습니다. 이 시점에서 csharpListObj
Python의 정수 배열이 있는 완전한 기능의 C# 배열입니다. 그리고 이를 증명하기 위해 콘솔에 모두 나열할 수 있습니다!
요약
결론적으로 C# .NET Core 애플리케이션 내에서 Python을 사용하는 것은 쉽고 원활합니다. Python for .NET은 Python 인터프리터와 상호 작용하고 Python 함수를 호출하기 위한 많은 메서드를 제공합니다. 이러한 방법을 사용하면 Python의 기능을 활용하여 C# .NET Core 애플리케이션에 새로운 기능을 추가할 수 있습니다. 무엇을 지을 건가요?!
특허
이 문서는 관련 소스 코드 및 파일과 함께 The Code Project Open License(CPOL) 에 따라 사용이 허가되었습니다.
광고 클릭에서 발생하는 수익금은 모두 웹사이트 서버의 유지 및 관리, 그리고 기술 콘텐츠 향상을 위해 쓰여집니다.